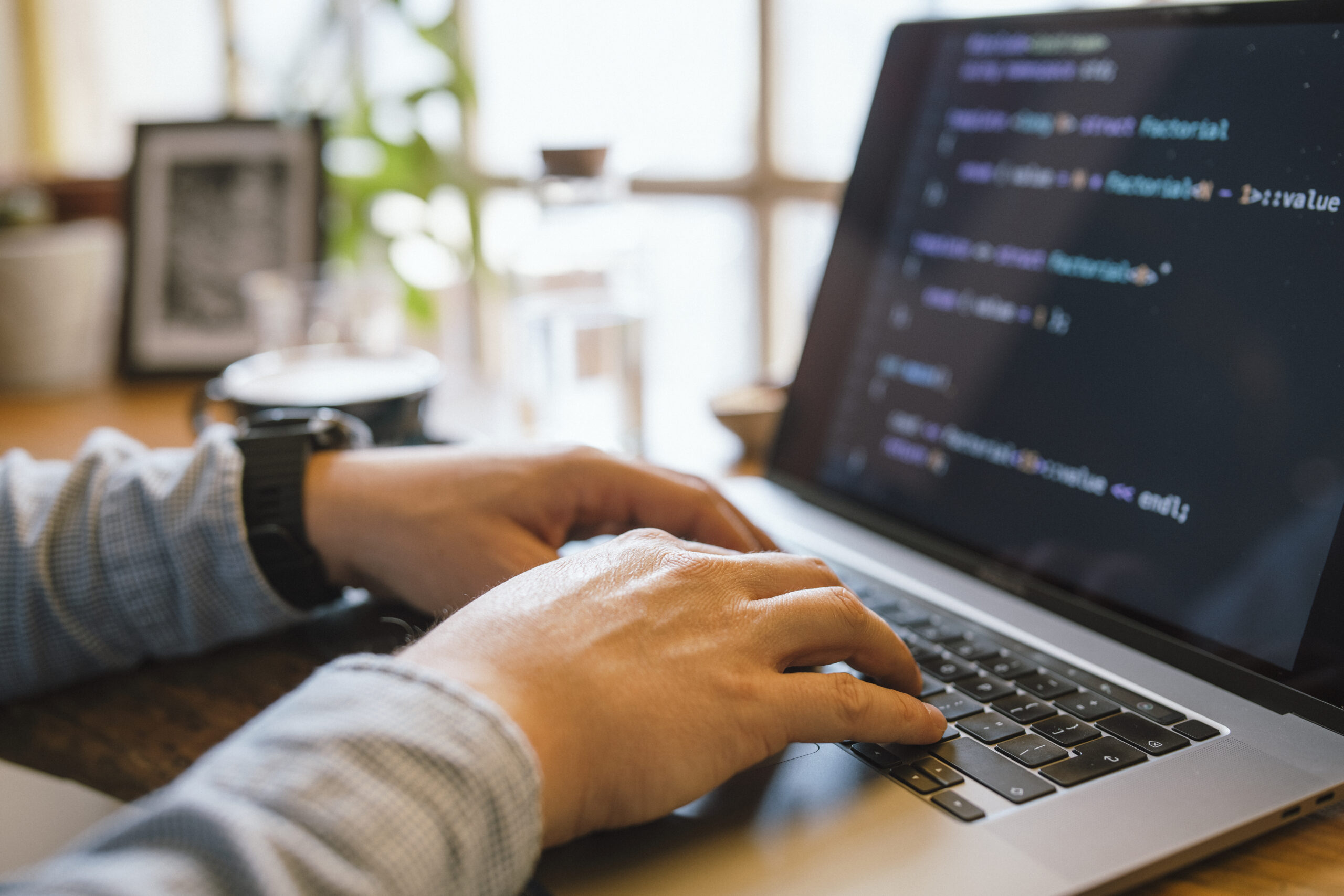
Debugging is One of the more essential — nevertheless generally missed — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go Improper, and Finding out to Assume methodically to unravel complications competently. Whether or not you're a newbie or even a seasoned developer, sharpening your debugging expertise can preserve hrs of disappointment and substantially boost your productiveness. Listed below are numerous techniques to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest methods builders can elevate their debugging techniques is by mastering the instruments they use every single day. Though producing code is just one Section of growth, realizing the best way to interact with it effectively all through execution is Similarly significant. Fashionable enhancement environments occur Geared up with highly effective debugging abilities — but a lot of builders only scratch the area of what these applications can perform.
Acquire, by way of example, an Integrated Enhancement Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools assist you to established breakpoints, inspect the value of variables at runtime, action by way of code line by line, and also modify code within the fly. When used the right way, they Enable you to notice particularly how your code behaves in the course of execution, which is priceless for monitoring down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for entrance-end developers. They allow you to inspect the DOM, keep an eye on network requests, watch actual-time general performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can convert irritating UI troubles into workable duties.
For backend or process-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage about running processes and memory management. Discovering these tools could have a steeper Mastering curve but pays off when debugging overall performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become relaxed with Model Command systems like Git to comprehend code heritage, obtain the precise moment bugs had been introduced, and isolate problematic variations.
Ultimately, mastering your resources implies heading over and above default options and shortcuts — it’s about producing an personal expertise in your development atmosphere to ensure that when concerns come up, you’re not dropped at nighttime. The higher you recognize your equipment, the greater time you may shell out resolving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
The most essential — and sometimes disregarded — measures in successful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, builders want to create a consistent ecosystem or circumstance the place the bug reliably appears. Without reproducibility, correcting a bug will become a match of likelihood, frequently bringing about wasted time and fragile code modifications.
The initial step in reproducing a challenge is gathering just as much context as you can. Inquire thoughts like: What steps led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the precise situations below which the bug takes place.
As soon as you’ve gathered ample info, seek to recreate the trouble in your neighborhood surroundings. This may suggest inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the sting conditions or state transitions included. These tests not just enable expose the issue and also prevent regressions Later on.
From time to time, the issue could be natural environment-certain — it would materialize only on particular working devices, browsers, or under certain configurations. Employing applications like virtual machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these types of bugs.
Reproducing the problem isn’t only a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continuously recreate the bug, you might be already halfway to fixing it. With a reproducible scenario, You can utilize your debugging equipment far more correctly, exam potential fixes safely, and communicate more clearly with your team or users. It turns an summary criticism right into a concrete challenge — Which’s where by builders prosper.
Read through and Recognize the Error Messages
Error messages tend to be the most respected clues a developer has when something goes wrong. Rather than looking at them as discouraging interruptions, builders must find out to treat mistake messages as immediate communications through the program. They frequently tell you what precisely took place, in which it happened, and sometimes even why it transpired — if you understand how to interpret them.
Begin by reading the information very carefully and in whole. Several developers, specially when below time pressure, look at the very first line and straight away start off creating assumptions. But further inside the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into engines like google — go through and understand them 1st.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it issue to a particular file and line range? What module or perform brought on it? These concerns can tutorial your investigation and stage you towards the responsible code.
It’s also beneficial to understand the terminology on the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can substantially accelerate your debugging course of action.
Some mistakes are obscure or generic, As well as in These situations, it’s very important to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date modifications inside the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede more substantial difficulties and supply hints about potential bugs.
In the end, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and turn into a more efficient and confident developer.
Use Logging Properly
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When used successfully, it provides real-time insights into how an application behaves, helping you comprehend what’s happening under the hood without needing to pause execution or step through the code line by line.
A good logging system starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for in-depth diagnostic information and facts all through progress, Details for standard activities (like productive begin-ups), Alert for probable troubles that don’t split the application, Mistake for genuine troubles, and FATAL when the procedure can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure critical messages and slow down your procedure. Target crucial events, state improvements, input/output values, and important final decision points in the code.
Format your log messages clearly and continually. Contain context, such as timestamps, ask for IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all with no halting This system. They’re Specifically valuable in creation environments where stepping by way of code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a very well-thought-out logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a technical activity—it's a sort of investigation. To effectively determine and correct bugs, builders will have to method the method just like a detective resolving a secret. This mindset assists break down intricate difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, collect as much relevant info as you are able to with out jumping to conclusions. Use logs, test cases, and user experiences to piece alongside one another a transparent photo of what’s going on.
Future, variety hypotheses. Check with you: What may very well be resulting in this habits? Have any alterations just lately been created towards the codebase? Has this problem happened right before underneath related situations? The objective is to slender down opportunities and recognize potential culprits.
Then, exam your theories systematically. Endeavor to recreate the trouble inside a managed natural environment. Should you suspect a specific purpose or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the results direct you closer to the reality.
Spend shut focus to small facts. Bugs usually hide from the least envisioned areas—similar to a missing semicolon, an off-by-a person error, or a race issue. here Be thorough and individual, resisting the urge to patch the issue devoid of totally being familiar with it. Short-term fixes may perhaps conceal the true problem, only for it to resurface afterwards.
Finally, retain notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future challenges and aid Some others understand your reasoning.
By contemplating similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed challenges in complicated techniques.
Generate Tests
Creating assessments is among the simplest ways to enhance your debugging capabilities and Over-all enhancement efficiency. Tests not just aid catch bugs early and also function a security Internet that offers you self-confidence when producing alterations on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly where and when a problem occurs.
Start with device checks, which deal with unique capabilities or modules. These compact, isolated checks can promptly expose no matter if a certain piece of logic is Functioning as anticipated. Whenever a test fails, you promptly know the place to seem, drastically lowering the time spent debugging. Device assessments are Specifically helpful for catching regression bugs—problems that reappear after Beforehand staying mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These enable be certain that different parts of your software perform together effortlessly. They’re notably helpful for catching bugs that manifest in advanced techniques with multiple parts or solutions interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and less than what situations.
Writing assessments also forces you to Consider critically about your code. To test a feature adequately, you'll need to understand its inputs, predicted outputs, and edge cases. This amount of understanding Obviously leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you are able to target correcting the bug and view your take a look at go when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the disheartening guessing sport into a structured and predictable approach—encouraging you catch much more bugs, more rapidly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s effortless to be immersed in the situation—gazing your screen for hours, attempting Remedy just after solution. But Probably the most underrated debugging resources is actually stepping absent. Getting breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new standpoint.
If you're far too near the code for far too very long, cognitive tiredness sets in. You would possibly start out overlooking evident glitches or misreading code that you just wrote just hrs previously. On this state, your brain becomes fewer economical at challenge-fixing. A short walk, a espresso crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report discovering the foundation of a challenge once they've taken time for you to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, In particular for the duration of for a longer time debugging classes. Sitting down in front of a monitor, mentally caught, is not just unproductive but also draining. Stepping absent permits you to return with renewed energy in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, extend, or do something unrelated to code. It could feel counterintuitive, In particular under restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, using breaks will not be a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a psychological puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each bug you face is a lot more than simply a temporary setback—It truly is a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can train you a little something valuable should you make time to replicate and review what went wrong.
Begin by asking oneself a number of critical thoughts as soon as the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit testing, code evaluations, or logging? The responses generally expose blind places with your workflow or knowledge and make it easier to Make more robust coding practices relocating forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or preserve a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you uncovered. With time, you’ll start to see patterns—recurring challenges or prevalent problems—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug along with your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast information-sharing session, assisting others steer clear of the identical issue boosts workforce effectiveness and cultivates a stronger Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers will not be the ones who publish perfect code, but individuals who continuously understand from their mistakes.
In the long run, each bug you correct provides a fresh layer on your skill set. So upcoming time you squash a bug, take a second to replicate—you’ll come away a smarter, additional able developer as a result of it.
Summary
Improving your debugging expertise usually takes time, practice, and persistence — although the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be better at Everything you do.